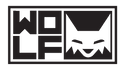

PROGRAMMING
I specialize in C++ and C# in game development settings. Compiled here are a number of scripts written for independent projects and demonstrations.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
Battle Manager
This C# script was created for Trinity. The script manages the flow of battle and makes use of coroutines and singletons to quickly and efficiently provide information to whatever components require data on the number of characters, enemies, and hazards that are present.
​
​
​
​
A full version of the script can be viewed through the GitHub link below.
1 /// <summary>
2 /// Spawns the selected attack prefab and starts it after a brief delay.
3 /// </summary>
4 public void Attack()
5 {
6 Battle_RangeMarker.KillAll();
7 if (directionSelection != null) StopCoroutine(directionSelection);
8 if (spaceSelection != null) StopCoroutine(spaceSelection);
9
10 if (Battle_UI.instance != null)
11 {
12 Battle_UI.instance.FadeDescription(0);
13 }
14
15 if (curAttack == null)
16 {
17 curAttack = Battle_UI.instance.SpawnAttack(FindAttackPrefab(selectedWeapon),
18 selectedPlayer.gameObject).GetComponent<iAttack>();
19
20 if (selectedWeapon.specialTactic == false) selectedPlayer.ChooseTactic();
21
22 Invoke("TriggerAttack", 2f); //delay so attack doesn't immediately start
23 }
24 }
Binary Search Tree
This C++ script was created as a programming exercise. It is a simple implementation of a binary search tree, where nodes are added to a list in order to format data for quick access.
​
​
​
​
​
​
​
A full version of the script can be viewed through the GitHub link below.
​
1 void bst::Report()
2 {
3 std::stack<Node*> myStack;
4 Node * tempNode = root;
5
6 while (tempNode != nullptr || !myStack.empty())
7 {
8 while (tempNode != nullptr)
9 {
10 myStack.push(tempNode);
11 tempNode = tempNode->leftChild;
12 }
13
14 tempNode = myStack.top();
15 myStack.pop();
16
17 tempNode->PrintWithLevel();
18 std::cout << std::endl;
19
20 tempNode = tempNode->rightChild;
21 }
22 }